
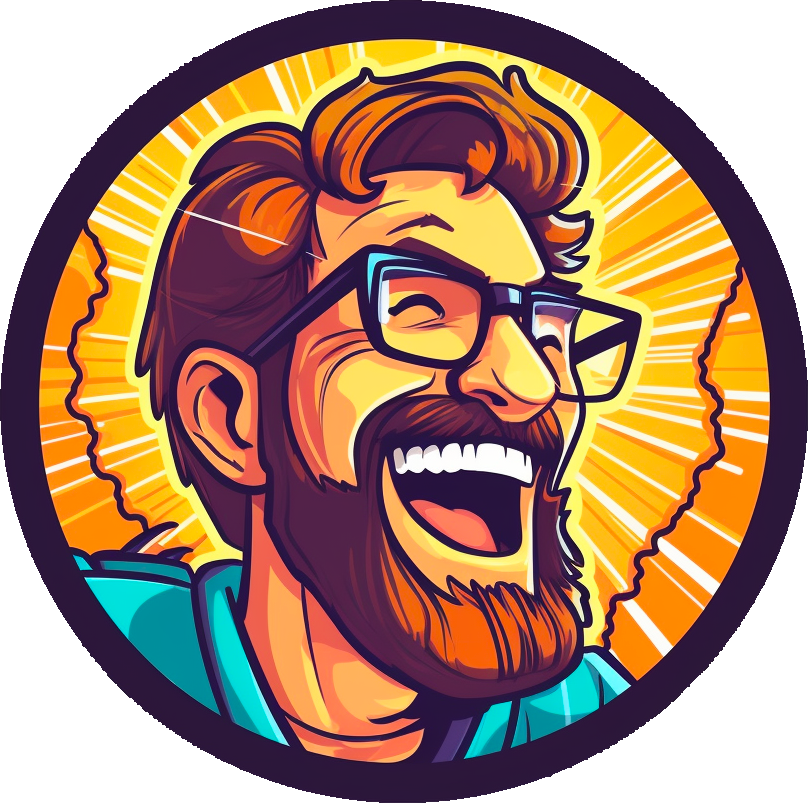
It’s a lacking point yes but unless you want to use a closed-source library it’s also a non-issue, which is why it has never been given priority. It’s not like language semantics would prevent portable dylibs it’s that there’s more important fronts to improve Rust on. A proper solution would take quite some engineering effort, and do note that C doesn’t have a proper solution either it just lets you link stuff up willy-nilly and then crash. Rust is actually in a better position to implement a proper solution than C is.
The “big project” thing is a red herring given that rust compiles incrementally. I know it is technically possible to not rebuild everything from scratch in C but the code has to specifically written to not break assumptions your build system makes while rust is happily re-using the compilation results for one function in a file while discarding those of another because actual dependencies are actually tracked. Out of the box.
Speaking of large Rust projects and proper type-safe linking: The WebAssembly folks are hashing out their Component Model which isn’t really limited to compiling to wasm, in principle: Big picture it’s a way to programmatically specify ABIs and even derive ABI translation code. That might be a good option as a rust-specific solution would be, well, rust-specific and when you engineer something that can support multiple versions of a language you can just as well engineer a bit more and have something cross-language.
They don’t. C compilers compile single files produced by the c preprocessor (resolving all
#include
s), they have no concept of multi-file projects. That’s a thing for the build system, such as make, and it needs dependency information from the preprocessor to do its job (cpp -M
), and once it has that it has to act correctly on them which is often completely broken because people don’t understand make. Like using it recursively, bad idea. In the wild, a random C project at work you’ll come across needs a full rebuild to build cleanly. Things have gotten better with things like cmake getting more popular but the whole thing is still brittle. GNU autohell certainly makes nothing better, ever.Everything will always have an ABI because ABI is just API in the target language, whatever that may be. If your program is compiled and can run it uses an ABI.
The core wasm abi is less capable than the C abi: You get scalar values and pointers, that’s it. No structs, no nothing, memory layout is completely unspecified. The component model allows compilers to say “so I’m laying out strings like this and structs like that” giving linkers a chance to say “yeah I can generate glue code between you two”.
C isn’t even close to being viable according to your standards people just have gotten used to the jank.
Rust doesn’t have portable dylibs precisely because it isn’t a hot mess. Because it’s actual work to do it properly. Unlike everyone else. Meanwhile It speaks the local C ABI fluently (they differ by architecture and operating system, btw), which isn’t a thing that can be said about many languages that aren’t C.
Differently put: What, precisely, do you want to do? Have you any actual use-case for your doubts, or are they spooks?