
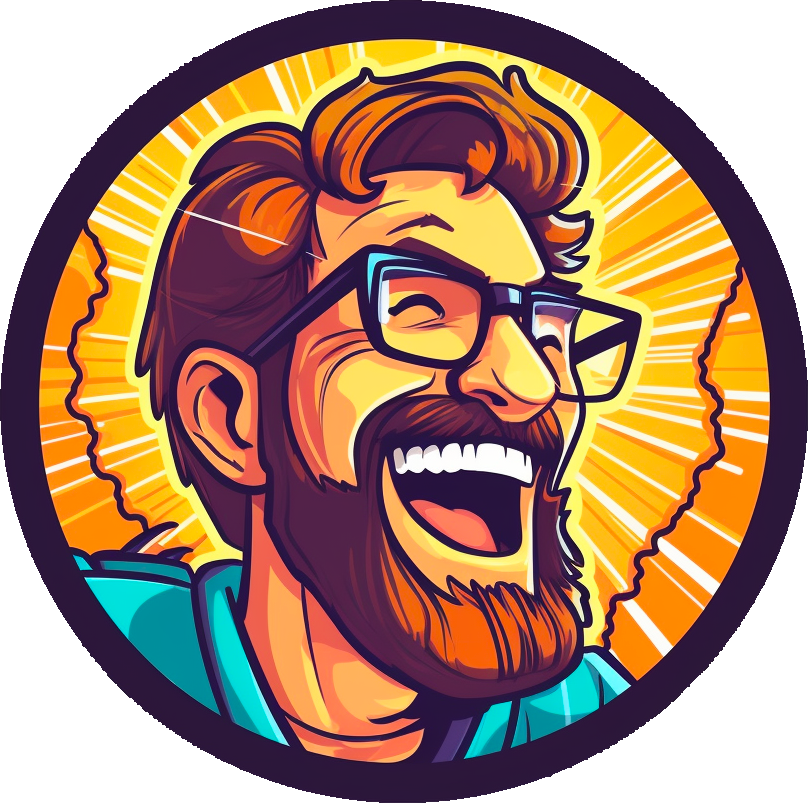
Anything that’s not an integer or a range doesn’t belong inside []
. Much more readable to use zip, map, filter, etc. And more powerful.
EDIT: that was meant for indexing lists. Strings inside []
for indexing ducts are fine.
Anything that’s not an integer or a range doesn’t belong inside []
. Much more readable to use zip, map, filter, etc. And more powerful.
EDIT: that was meant for indexing lists. Strings inside []
for indexing ducts are fine.
I haven’t used npm. But pip is horrible. Some times I’ve used a well-known library that only works on linux, but there is no mention of it whatsoever, and it installs without problem. The error only happens at run-time (not even when importing!) and says nothing about platform-dependency. I only learned that it was a linux-only library because I happened to try running it on a Linux machine to see if it worked.
Many times you have to set up your environment a specific way (environment variables, PATH, install dependencies outside of pip) for it to work, and there’s no mention of it anywhere. Sometimes you install the library with pip, sometimes with apt, and there is no way to know which one. And sometimes the library is both in apt and pip, but the pip one does nothing.
Furthermore, good luck importing a library. You might have installed it with “pip install my-library” but to import it you have to do “import MyAwesomeLibrary3”. And pip won’t tell you about that.
The mistake was choosing a language, and afterwards searching for a use to the language you just learned.
I don’t think changing a profession’s terms to “prevent stupid jokes” is a smart move.
Iirc Ubuntu names their home files “Downloads”, “Documents”, and so on. Same with windows (there are a lot of uppercase letters in windows files). I’ve had issues with Cargo.toml before. And not just cargo, many config files use case to signal priority (so if both Makefile and makefile exist, Makefile will be used (or other way around)). Downloaded files are a gamble. Files created by user input (so for example if I wanted my user to be “Calcopiritus”, my home would be “/home/Calcopiritus”.
Uppercase letters might not be common in filenames, but they are not nonexistent.
I believe the game was 10 days old when they shut it down. There are no concord fans. You can’t have fans in 10 days.
My guess is that they knew it was going to be a shit game, but realized too deep in the development phase. So they just released it as soon as possible and didn’t waste more money on it (marketing). My guess is that the released it instead of cancel just in case they were wrong and people actually liked it.
They are not created by people. They are created by programs.
I can make MY files all lowercase, but 99.999% of files on my computer are not created by me. And some of them have capital letters.
When you say "canse insensitive file*, do you mean lowercase files? Or is there an option?
Idk why we talking about mouses. When I’m on Linux, most of the time it’s through ssh.
This is the first time I’ve seen uppercase denoting scope. Usually it is done with a “_” or “__” prefix.
Casing styles usually mean different identifier types.
snake_case or pascalCase for functions and variables, CamelCase for types, UPPER_SNAKE_CASE for constants, and so on.
If we want to apply this to file systems, you could argue something like: CamelCase for directories, snake_case for files, pascalCase for symlinks, UPPER_SNAKE_CASE for hidden files.
I’m with windows on this one. Case insensitive is much more ergonomics with the only sacrifice represented by this meme. And a little bit of performance of course. But the ergonomics are worth it imo.
This is perfectly fine to do under GPL as well. I don’t see the problem.
There are companies that sell Linux distros on a DVD or USB drive.
I don’t think they do actual games for kids anymore, they are money-traps most of the time.
I’d search instead for old console games and play them on an emulator on Android.
It might be very confusing for a child if she has never played on a console though, since no touchscreen support and having buttons on the screen instead.
There are only 2 reasons I would buy a motherboard:
What feature would make you upgrade just the motherboard? Especially given how expensive they are nowadays (sometimes even more expensive than the CPU).
Hardware doesn’t need to be too weird. Back when I bought my laptop, it was a kinda recent model so most of its features didn’t work in Ubuntu (I say Ubuntu because it’s the distro that worked best. Tried many others and they had even worse support). After a year or so it worked mostly, except some things.
To this day, 4 years later, the display brightness control still doesn’t work correctly.
I don’t think hiding the problems do any good. The Linux desktop/laptop experience is not good, specially for non-programmers. It’s usable, but not good.
That was a feature they removed.
Some WebApps are only available as websites and don’t have a native counterpart.
I’d personally like this feature to come back so I can use those WebApps without having to have other browser-things clutter the UI (like multiple tabs, url bar, etc.) and aren’t needed when you only want to open one webapp.
It’s actually more confusing/less correct to close bodyless elements in HTML. Since HTML treats “/>” the same way as “>” which could lead to a “/>” tag not actually being closed, if it is used on a non-selfclosing tag.
That is because when you’re a beginner, you read everywhere that you should be using anaconda and jupyter notebooks. I know because I did so. Neither of them lasted more than a week on my computer though.